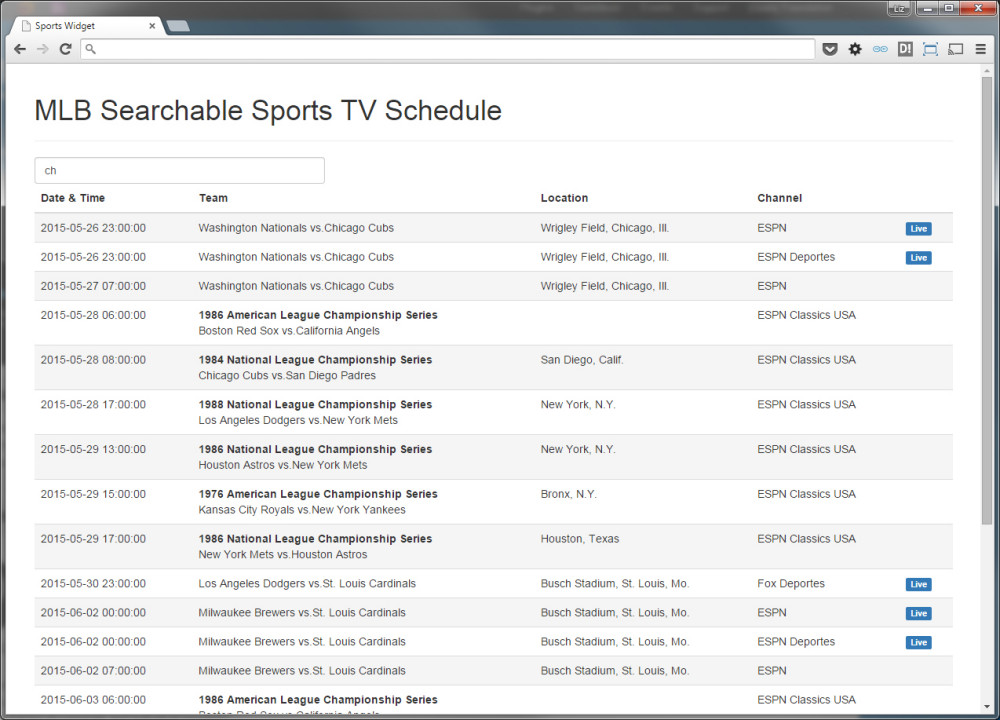
Before we begin, you will need your API key. If you don’t have a key, sign up for an API account at developer.tvmedia.ca. For this little project, we’re going to use Bootstrap, jQuery and the jQuery-Searchable Plugin.
In order to make an API call, we need to build the API call. The call is made up of several parts.
- API Key – Secret key used to access APIs
- Base URL – http://api.tvmedia.ca/tv/v4/
- Lineup ID – Every call requires a lineup ID. A lineup is a collection of stations through a TV provider.
- API Method – The operation we’ll use to access TV listings.
- League ID – We’re using MLB, but you can use any league(s).
- End Date – The amount of future programming to get, in our case 15 days but plans vary.
$secretKey ='&api_key=YOURAPIKEY';
$apiURL = 'http://api.tvmedia.ca/tv/v4/';
$lineupID = '36617';
$apiMethod = 'lineups/'. $lineupID .'/listings?';
$leagueFilter = '&league=MLB';
$endDate = date("Y-m-d", strtotime("+15 days"));
Now that we’ve set up all the variables, we can build the URL variable to make the call.
$url = $apiURL . $apiMethod . $leagueFilter . $secretKey . '&end=' .$endDate;
If you echo $url you will see the request URL.
http://api.tvmedia.ca/tv/v4/lineups/36617/listings?&league=MLB&api_key=YOURAPIKEY&end=2015-06-10
Now that we’ve got our request URL, it’s time to get the JSON data and convert the data to an array that we’ll use in our sports schedule.
$file_contents = file_get_contents($url);
$listings = json_decode($file_contents, true);
If you’d like to see the values of what you’ve returned you can print out the information with
print_r($listings)
Now that we have all the data we need, let’s build the sports schedule. Again, we’re using Bootstrap, jQuery and jQuery-Searchable Plugin.
<div class="container">
<div class="row">
<div class="row">
<div class="col-lg-12">
<div class="page-header">
<h1>MLB Searchable Sports TV Schedule</h1>
</div>
</div>
</div>
<div class="row">
<div class="col-lg-4">
<input type="search" id="search" value="" class="form-control" placeholder="Search for your teams or venue">
</div>
</div>
<div class="row">
<div class="col-lg-12">
<table class="table" id="table">
<thead>
<tr>
<th>Date & Time</th>
<th>Team</th>
<th>Location</th>
<th>Channel</th>
<th></th>
</tr>
</thead>
<tbody>
<?php foreach ($listings as $listing) { ?>
<tr>
<td><?php echo $listing['listDateTime'];?></td>
<td><?php if ($listing['event']) {
echo '<strong>'.$listing['event'].'</strong> <br>';
}?>
<?php if ($listing['team1'] != '') {?>
<?php echo $listing['team1'];?> vs.<?php echo $listing['team2'];?>
<?php } else echo "Teams To Be Announced"; ?>
</td>
<td><?php echo $listing['location'];?></td>
<td><?php echo $listing['name']; ?> </td>
<td>
<?php if ($listing['live'] == 'true') {?>
<span class="label label-primary">Live</span>
<?php } ?>
</td>
</tr>
<?php } ?>
</tbody>
</table>
</div>
</div>
</div>
<script>
$(function () {
$( '#table' ).searchable({
striped: true,
oddRow: { 'background-color': '#f5f5f5' },
evenRow: { 'background-color': '#fff' },
searchType: 'default'
});
$( '#searchable-container' ).searchable({
searchField: '#container-search',
selector: '.row',
childSelector: '.col-xs-4',
show: function( elem ) {
elem.slideDown(100);
},
hide: function( elem ) {
elem.slideUp( 100 );
}
})
});
</script>
Now we have a basic webpage that displays upcoming MLB baseball games and the channel users can find the game.
You can download this entire project.
Do you like this project and want to see more or just have some questions? Leave us a note in the comments!